Drip Irrigation Control System v4.0 – Web Server
In the past, I explained the hardware and software parts of the system. This concluding post on my drip irrigation control system will go into the details of the web server. As you know, I wanted to build a system that I can control from anywhere. In the previous designed, I had to be near the control system to configure the drip time and duration using buttons on the board.
Sometimes I am on the terrace and want to pause or start the drip to adjust something. However, the control system is on the ground floor. Each time I need to reconfigure, I have to run up and down two flights of stairs. Certainly good physical activity, but I prefer a simpler method. That is when the idea of a network controlled system came to my mind. And what better way than to run a web server on the device and configure using the web frontend?
Web Server on ESP8266
You already know from my previous posts that I am using LoLin NodeMCU v3 board. That board comes with ESP8266 which can run a web server. As described in my previous post, I have already setup the SPI file system on the device. The plan is to serve html files from that space.
void setup() { Serial.println("[WebServer]: Setting up server"); server.on("/", HTTP_GET, [](AsyncWebServerRequest *request) { request->send(SPIFFS, "/index.html", "text/html"); }); server.serveStatic("/", SPIFFS, "/"); server.begin(); Serial.println("[WebServer]: Server started"); }
I used WebSocket instead of POST or GET methods for client-server communication. The reason was that if there are multiple clients connected to the server, all of them could be updated via WebSocket when one of the client updates the state of the device.
void setup(WebSocketListener* webSocketListener) { this->webSocketListener = webSocketListener; webSocket.onEvent( [this](AsyncWebSocket* server, AsyncWebSocketClient* client, AwsEventType type, void* arg, uint8_t* data, size_t len) { this->onWebSocketEvent(server, client, type, arg, data, len); }); server.addHandler(&webSocket); server.begin(); Serial.println("[WebSocket] Web socket server started"); // Add service to MDNS-SD MDNS.addService("sprinkler", "udp", port); Serial.println("[Websocket] Service added to mDNS"); }
As an example for client-server communication, lets says I am connected to the control system's web server from both my laptop and mobile device. Then, when I turn on the drips from the laptop, the message to turn on is sent from the laptop to the web server. The control system turns on the drips and the state is updated. Next, it turns around and updates all the WebSocket clients that are connected to it with the latest state. So both the laptop and phone get notified of the new state and can update their UI with the new information. Check out the video at the end of the post to understand my point.
Web Frontend
For the web frontend code, I decided to go with Open Web Components project. It is quite heavy weight for a small board like ESP8266. But the reason I wanted to go with it is because I wanted a good development environment. The plan was to compile the code to minimal html and JS. Then copy those files into the SPIFFS space from where ESP8266 will just serve the static files. I upload the files to ESP8266 over-the-air. With this setup, I can use all the nice UI elements to make the web page look pretty.
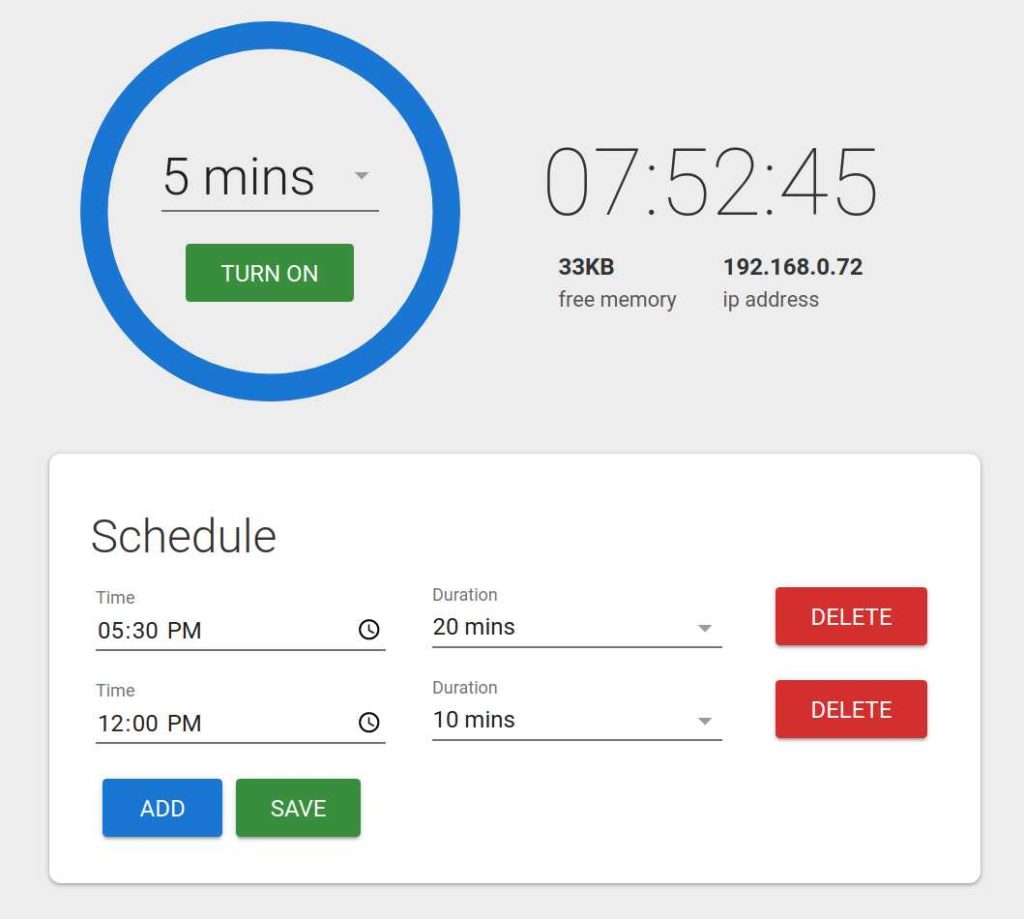
The best part is that I can develop the web frontend independently of the control system code. NodeJS is an excellent way to build high quality web apps. If you are interested in the web frontend code, check out my github repo.
Conclusion
This concludes the topic of drip irrigation control system. The main advantage of the control system is that it relieves us of the mundane task of watering the plants everyday. Moreover, when we are travelling, the plants still get watered. And finally, now I can control the system from anywhere :). I am still looking forward to building v5.0 with humidity sensor. That way, when there is rain, the drips won't start on schedule if the soil is still wet. In the mean time enjoy the demo of the control system's web socket communication which keeps all the clients and ESP8266 in sync.